Solving a Pair Sum Problem (Step by Step)
Whiteboard interviews can be intimidating, but they’re an essential part of the hiring process for developers. They challenge you to think on your feet, solve problems, and write clean code—all without the help of your IDE. The key to mastering them is to practice solving problems step by step, breaking them down into smaller tasks, and understanding the tools and data structures available to you.
In this article, we’ll walk through a pair sum problem from a whiteboard interview, following a structured approach. Along the way, I’ll show you the exact thought process and tools I used to solve it. By the end, you’ll also learn some important TypeScript/JavaScript concepts like Sets, generators, for…of loops, and more!
Why Whiteboard Interviews Matter
Whiteboard interviews aren’t just about solving complex problems quickly. They’re also about communication, clarity, and showing how you approach a challenge. In a real-world scenario, most of the time you’ll be working in teams, and being able to explain your thought process is just as valuable as getting the correct answer.
Alright, enough talk—let’s jump into the problem-solving mode!
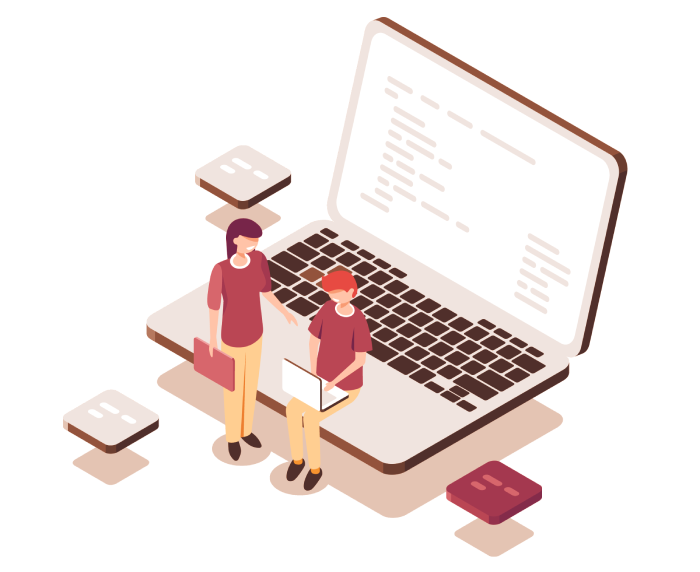
The Problem: Find a Pair that Sums to a Given Number
Here’s the problem we’re tackling:
- You are given an array of numbers and a target sum.
- Your task is to determine if any two numbers in the array sum up to the target number.
[1, 2, 3, 9]
withsum = 8
should returnfalse
(no pair sums to 8).[1, 2, 4, 4]
withsum = 8
should returntrue
(because4 + 4 = 8
).
For example:
Simple, right? Well, we need to go step by step to ensure our approach works in different scenarios.
Methodology: REACTO
To tackle this problem, I’ll be using a popular interview method called REACTO. Here’s how it breaks down:
- R – Repeat the Question: Clarify the problem to avoid misunderstandings.
- E – Example: Think of test cases to fully understand the expected behavior.
- A – Approach: Discuss different ways to solve the problem, comparing time and space complexity.
- C – Code: Start writing the solution based on your chosen approach.
- T – Test: Run test cases to verify if the code works.
- O – Optimize: Refine your solution for better performance if possible.
Step 1: Repeat the Question
The first thing to do is repeat the problem to make sure we’ve captured all the important details:
- Input: An array of integers and a target sum.
- Output: Return
true
if there’s a pair of numbers whose sum equals the target, andfalse
if not.
We also clarified a few extra points:
- Should we return the pair or just a boolean? (Boolean:
true
orfalse
). - Should the solution handle unordered arrays and negative numbers? (Yes).
- What happens if there are no pairs? (Return
false
). - If there are multiple valid pairs, we only need to find one.
Step 2: Example
Let’s test the understanding with two examples:
- For the array
[1, 2, 3, 9]
and sum8
:No two numbers sum to
8
, so we returnfalse
. - For the array
[1, 2, 4, 4]
and sum8
:4 + 4 = 8
, so we returntrue
.
Now that we have clarity, we can move to the approach.
Step 3: Approach
There are a couple of approaches to solving this problem:
Brute Force (O(n²)):
We could try every possible pair of numbers in the array and see if their sum equals the target. This would involve using two loops—one nested within the other—to test every combination of numbers. This approach works but is inefficient because it has a time complexity of O(n²).
Optimized Approach (O(n)):
A better solution would be to use a HashSet (in JavaScript, it’s called a Set
). This lets us keep track of numbers we’ve seen as we iterate through the array. Here’s the plan:
- For each number in the array, calculate the complement (target sum – current number).
- Check if that complement is already in the
Set
. - If it is, return
true
because we’ve found a pair. - If not, add the current number to the
Set
and continue. - If we finish iterating without finding a pair, return
false
.
This solution only requires a single pass through the array (O(n)) and uses extra space (O(n)) for the Set
.
Step 4: Code
Let’s write the optimized solution:
function hasPairWithSum(arr: number[], sum: number): boolean {
const seenNumbers = new Set<number>();
for (let num of arr) {
const complement = sum - num;
if (seenNumbers.has(complement)) {
return true; // Found a pair that sums to the target
}
seenNumbers.add(num); // Keep track of the current number
}
return false; // No valid pair found
}
// Test cases
console.log(hasPairWithSum([1, 2, 3, 9], 8)); // Output: false
console.log(hasPairWithSum([1, 2, 4, 4], 8)); // Output: true
This solution is efficient and works well for arrays of any size. Now let’s expand the problem a bit…
Step 5: Handling Infinite Arrays (Streaming Data)
What if the array isn’t finite, or we’re dealing with streaming data? We can’t store all the elements of an infinite array, but we can still process the data as it comes in. In this case, we use generators and iterables to handle the data in real-time.
Here’s how you can solve the same problem with streaming data:
function* infiniteNumberStream() {
let i = 0;
while (true) {
yield i++; // Generates numbers infinitely
}
}
function hasPairWithSumStream(stream: Iterable<number>, sum: number): boolean {
const seenNumbers = new Set<number>();
for (let num of stream) {
const complement = sum - num;
if (seenNumbers.has(complement)) {
return true;
}
seenNumbers.add(num);
}
return false;
}
// Example with a simulated infinite stream
console.log(hasPairWithSumStream(infiniteNumberStream(), 10)); // Output: true (5 + 5 = 10)
In this case, the generator (infiniteNumberStream
) keeps producing numbers indefinitely, and we process them one at a time without needing to store all of them in memory.
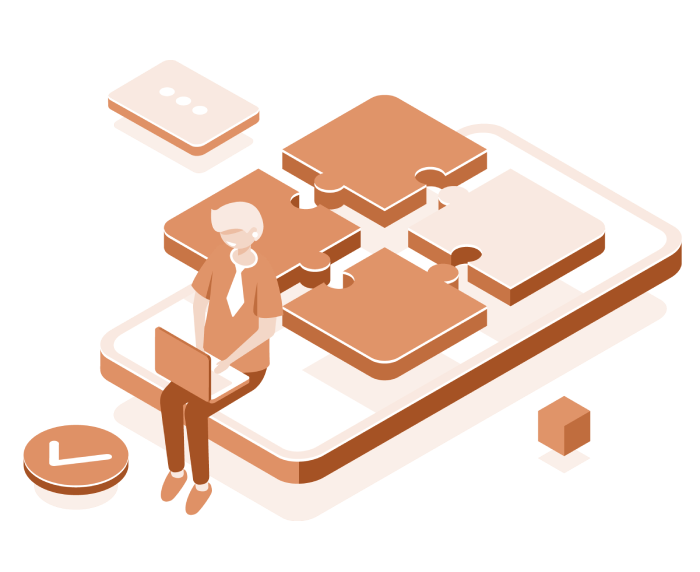
Step 6: Understanding the Tools Used
Understanding Key Angular Concepts Through JavaScript/TypeScript
Hey everyone! Today, we’re diving into some essential concepts in JavaScript and TypeScript that are super useful in Angular development. Let’s break it down step by step!
1. Set
in JavaScript/TypeScript
What is it?
A Set is a data structure that stores unique values. Unlike arrays, Sets
don’t allow duplicates, which makes them handy when you want to quickly check if you’ve seen a value before.
How do we use it?
In our code, we use a Set
to store all the numbers we’ve seen during an array iteration. This lets us quickly check if the complement of a number has been seen, helping us find pairs that sum to a target value.
Creating and using a Set
:
const seenNumbers = new Set(); // Create a Set for numbers
// Adding values to the Set
seenNumbers.add(5); // Now the Set contains the number 5
// Checking if a value is in the Set
if (seenNumbers.has(5)) {
console.log("The number 5 has been seen!");
}
// Removing values from the Set (not used in our code, but good to know)
seenNumbers.delete(5);
Why use a Set
?
Sets
allow for fast check and insert operations in O(1) time, making them efficient for large data sets.
2. for...of
Loop
What is it?
The for...of
loop is used to iterate over the values of an iterable object (like arrays, sets, or even streams of data). It allows us to loop through elements without needing indexes (unlike traditional for
loops).
How do we use it?
In our code, we use for...of
to iterate over the numbers in an array or stream, processing one number at a time.
Simple example:
const arr = [1, 2, 3, 4];
for (let num of arr) {
console.log(num); // Prints 1, 2, 3, 4 on each iteration
}
In our code, we apply it like this:
for (let num of arr) {
// Process the current number 'num'
}
3. Generator Functions
What is it?
Generator functions are a way to create functions that can be paused and resumed. They return an object called an iterator that produces values “on demand,” one at a time.
- A
generator
uses theyield
keyword to return incremental values. - The function doesn’t generate all values at once; it pauses at
yield
and resumes when needed. This is great for simulating infinite arrays or continuous data streams.
How do we use it?
In our code, we create a generator function to simulate an infinite stream of numbers, showing how we might handle continuous data.
Simple generator example:
function* numberGenerator() {
yield 1; // Yields the value 1
yield 2; // Yields the value 2
yield 3; // Yields the value 3
}
// Using the generator
const gen = numberGenerator(); // Creates the iterator
console.log(gen.next().value); // Output: 1
console.log(gen.next().value); // Output: 2
console.log(gen.next().value); // Output: 3
Each time we call next()
, the generator advances to the next yield
and returns that value. This gives us granular control over iteration.
In our code:
function* infiniteNumberStream() {
let i = 0;
while (true) {
yield i++; // Yields infinite numbers starting from 0
}
}
This function generates infinite numbers one by one, without needing to store them all at once. Instead of creating an array of infinite numbers, we create a continuous stream that provides values as needed.
4. Iterables and Iterators
What are they?
An Iterable is an object that can be looped over, like arrays or strings, or any object implementing the [Symbol.iterator]()
method. It defines how an object can be iterated.
An Iterator is an object that keeps track of the current position in the sequence and knows how to get the next value.
How do we use it?
The for...of
loop works with iterables (like arrays and generators). In the case of arrays, the iterable is predefined, but for infinite streams, we use a generator
to manually create the iteration behavior.
Example of Iterables:
const arr = [10, 20, 30]; // An array is an iterable
for (let num of arr) {
console.log(num); // Prints 10, 20, 30
}
In the case of the generator
, it also creates an iterable that can be consumed in a for...of
loop.
5. Functions and Typing in TypeScript
Functions in TypeScript:
Defining functions in TypeScript is similar to JavaScript, but we can add types for more safety and to avoid common errors.
How do we use it?
In our examples, we define functions with types for the parameters and return value.
Simple function example in TypeScript:
function sum(a: number, b: number): number {
return a + b;
}
console.log(sum(2, 3)); // Output: 5
Here we specify that a
and b
are of type number
and that the function returns a number
.
In our code:
function hasPairWithSumStream(stream: Iterable, sum: number): boolean {
// The function takes an 'iterable' of numbers and a target number, returning a boolean
}
stream: Iterable<number>
: Thestream
parameter is of typeIterable<number>
, meaning it can be looped over (could be an array, a generator, etc.).sum: number
: The target sum.: boolean
: The function returns aboolean
, eithertrue
orfalse
, depending on whether we found the pair.
Recap of Concepts You Learned:
Set
: A data structure that stores unique values and allows for efficient searching.for...of
Loop: A simple loop for iterating over arrays or iterable objects.- Generators: Functions that can yield values on demand, useful for continuous data streams or large datasets.
- Iterable and Iterators: Concepts for objects that can be traversed item by item.
- TypeScript Typing: Ensuring safety and predictability in code with type definitions.
In this article, we walked through a typical whiteboard interview problem and learned:
- The importance of breaking down a problem using structured methodologies like REACTO.
- How to implement an efficient solution using a Set to track seen numbers.
- How to handle more advanced scenarios like infinite streams using generators.
- The usage of common JavaScript/TypeScript features like
Set
,for...of
, andgenerators
to solve real-world problems.
When preparing for whiteboard interviews, it’s essential to focus on clarity, communication, and having a solid grasp of the basic tools available in the language you’re using.
Good luck with your interviews, and happy coding! 💻🚀
Sorry, the comment form is closed at this time.